1. Why am i writing such a document?
If you are searching
for a paper about minimaps on the internet, you won't really find much.
Maybe you find some informations, but they are really rare. So that
others don't have to search long i am providing some basics on that
topic.
2. What are minimaps actually and what is this paper about?
Minimaps are small
maps you can find mostly in rts games like AoE II or C&C. These
present the map in a little window mostly located in the interface.
Normally you don't find only the raw map on it, as the ground and the
environment, what won't be very useful, but also units, the fog of war
and maybe missiontargets.
I will refer to minimaps, that concern tilebased maps, in this paper.
There is also a sourcecode, written in C/C++ with use of the SDL, at the end of this doc that is
based on this theory. Here are a few examples of minimaps:
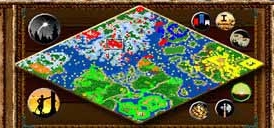
Minimap aus Age of Empires II
|
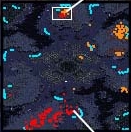
Minimap aus Starcraft
|
3. How do i make a minimap?
Basically a minimap
is done like a tilebased map and consists of an array of tiles
respectively references on tiles. If the map has several layers, you
have to consider that during the creation of the minimap.
That array consists mostly of numbers or chars, in the least cases you
will save there tiles so graphicdata directly in the map. So the map is
in an array. Now to the minimap. At first we have to make clear how it
is build up.
Like the real map it should consist of an array, that has the same size
as the normal map. That array don't consist of references to tiles but
values of the pixelcolor. But how do you find the data of that array?
And where does that color come from?
Quite simple. During the initialisation of the map, some people call it
loading, you have to calculate the average color of a tile. You have to
do that for every tile and than save it in the above-named array.
In the most cases you want your minimapsize to be constant. Besides i
would propose to have a standard mapsize. Bigger maps than these,
respectively smaller, will just be multiplicated ord divided by 2 during
the finding of the average pixelcolor.
That clearly means that at smaller maps you take four, eight or sixteen
pixels for a tile. And for bigger maps you take more tiles for each
average color. That way your minimapsize will stay constant. Well
capable cardsizes would be 64x64, 128x128 or 256x256.
Let us merge that. Our minimap will consist of an array for a constant
map, that includes values for colors of tiles. If the mapsize ist bigger
or smaller we have to consider that during the calculation of the
colorvalues.
4. How do i find the average colorvalue?
Well, how do we now
find out the colorvalue that our pixel in the minimap needs. That isn't
so hard, let's take a 32x32 pixel tile ( 64x32 or others work, too ).
Everyone knows how to calculate an average, you add all colorvalues of
the pixel together and divide with the number of pixels. That's it? Yes,
that's it.
But you have often colorvalues like that 0x452365. You shouldn't simply
add that kind of values together. Most suitable is to filter the r,g and
b values out, add these and finally divide these. If your map is bigger
you should add together all pixels of all tiles and of course divide
through the number of pixels of all tiles. At first here is some code
that works for one tile:
colorvalue getaverageColorvalue( picture )
{
r, g, b = 0;
numberOfPixels = picture.width * picture.height;
for( i = 0; i < numberOfPixels; i = i + 1 )
{
r = r + picture.colorvalue[ i ].R;
g = g + picture.colorvalue[ i ].G;
b = b + picture.colorvalue[ i ].B;
}
r = r / numberOfPixels;
g = g / numberOfPixels;
b = b / numberOfPixels;
return colorvalue( r, g, b );
}
That shouldn't be so hard to understand. The function is implemented
one more time in the C/C++ example code.
5. Ok, i have the colorvalue, what now?
Well, what you do
further on is up to you. There are several methods what you can do, e.g.
put the colorvalues in an array or make a minimap class with that data.
Or you just give each tile their color and make a drawroutine.
6. Example code
Here you can get an
example programm, that draws an minimalistic tilebased map that is
10x10 tiles sized. In the right is a 10x10 pixel-sized minimap shown.
The program was written in C/C++ with use of the SDL and SDL_image.
And that is how it looks:
|